DroidCo. Final
Posted: December 12, 2019 Filed under: Uncategorized Leave a comment »For the final stage of my project, I finished the display and game logic of DroidCo. As a final product, DroidCo. allows two players to use OSC to “write code” (by pressing a predetermined selection of buttons) that their droids will execute to compete. The goal of the game is to convert every droid on the grid onto your team.
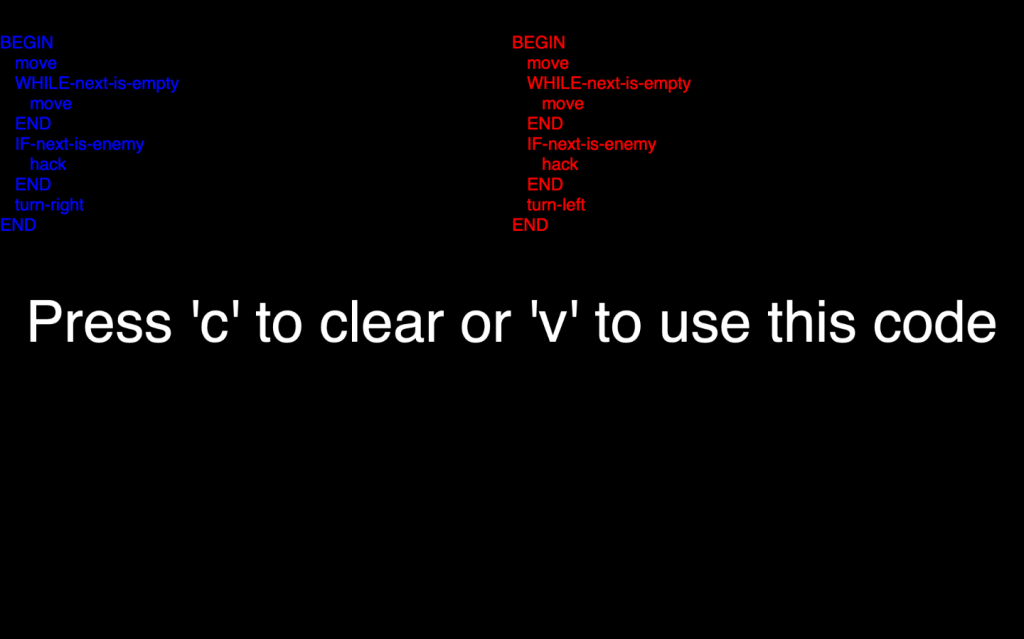
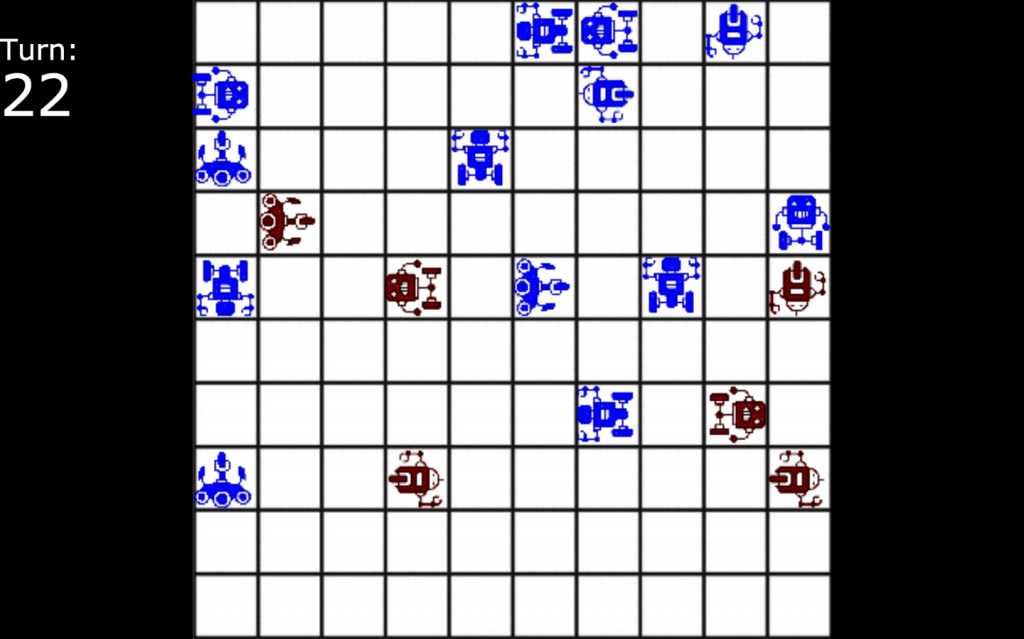
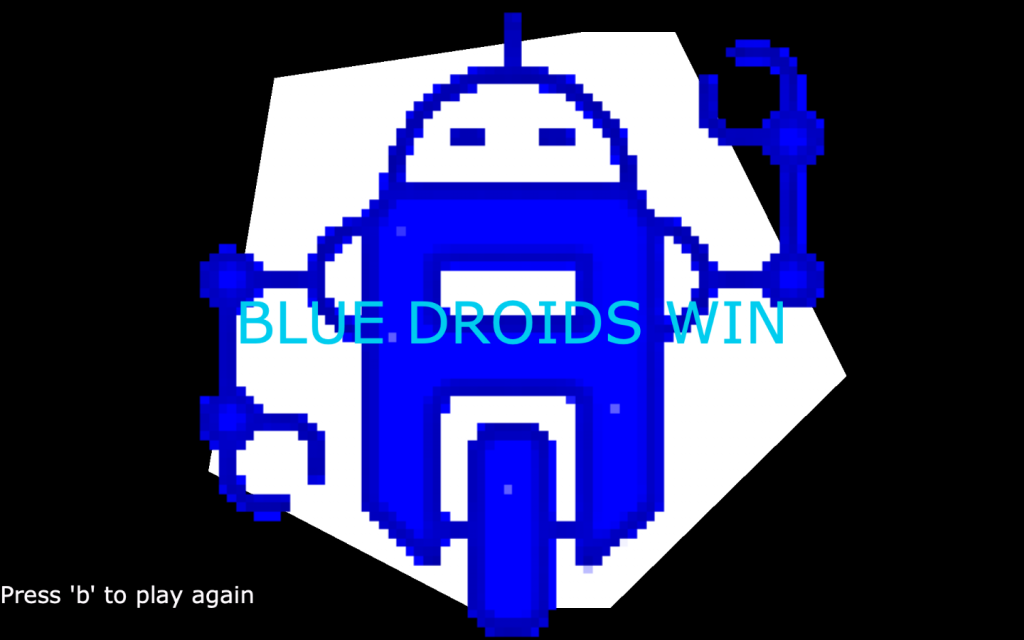
function main()
{
var worldState = JSON.parse(arguments[0]);
var droid = arguments[1];
var startIndex = (droid * 6) + 2;
var team = worldState[startIndex + 1];
var x = worldState[startIndex + 2];
var y = worldState[startIndex + 3];
var dir = worldState[startIndex + 4];
var red;
var blue;
var hor;
var ver;
var rot;
switch (team){
case 1:
blue = 50;
red = -100;
break;
case 2:
blue = -100;
red = 50;
break;
}
switch (x){
case 0:
hor = -45;
break;
case 1:
hor = -35;
break;
case 2:
hor = -25;
break;
case 3:
hor = -15;
break;
case 4:
hor = -5;
break;
case 5:
hor = 5;
break;
case 6:
hor = 15;
break;
case 7:
hor = 25;
break;
case 8:
hor = 35;
break;
case 9:
hor = 45;
break;
}
switch (y){
case 0:
ver = 45;
break;
case 1:
ver = 35;
break;
case 2:
ver = 25;
break;
case 3:
ver = 15;
break;
case 4:
ver = 5;
break;
case 5:
ver = -5;
break;
case 6:
ver = -15;
break;
case 7:
ver = -25;
break;
case 8:
ver = -35;
break;
case 9:
ver = -45;
break;
}
switch(dir){
case 0:
rot = 180;
break;
case 90:
rot = 270;
break;
case 180:
rot = 0;
break;
case 270:
rot = 90;
break;
}
return [red, blue, hor, ver, rot];
}
Above is the code for the “droid parser” which converts each droid’s worldState data into values that Isadora can understand to display the droid.
Overall, the game was a major success. It ran with only minor bugs and I accomplished most of what I wanted to do with this project. The code was rather buggy (I underestimated the strain new users could put on my code). I also ran into several problems with players’ code not executing exactly as they wrote it. These bugs are definitely due to the String Constructor (Isadora does not handle strings very well), but I think I can work out the kinks.